目次
桜を降らせるDEMO
JSとCSSで桜を降らせるエフェクトを実装しました。
まずは実装内容を確認しましょう、DEMOページをご覧ください。
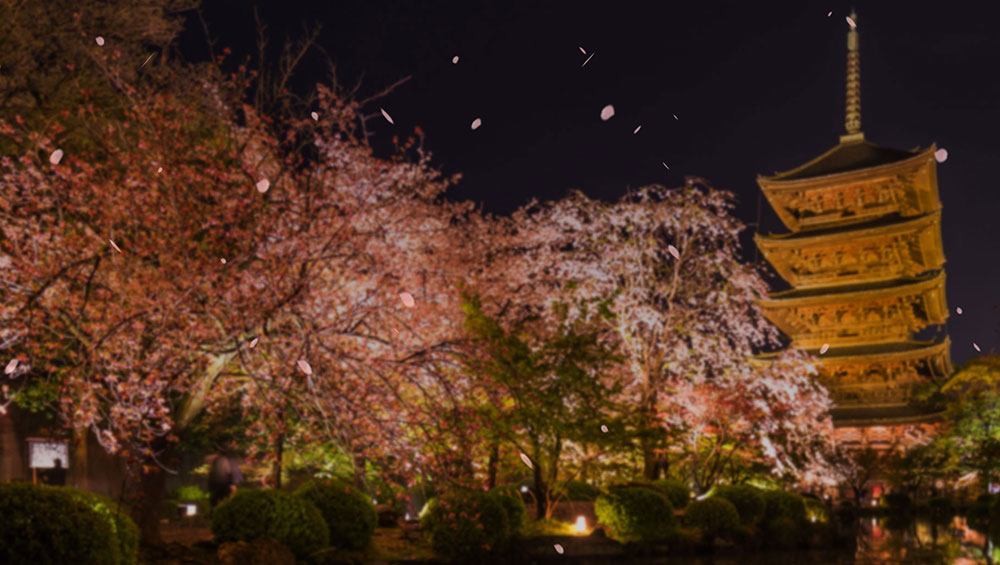
コピペ用実装コード
index.html
style.css
script.js
を作成し、以下のコードを貼り付けてください。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<title>JavaScript桜を降らせる</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<canvas id="effectimg" width="2000px" height="1000px"></canvas>
<script src="script.js"></script>
</body>
</html>
body {
margin: 0 auto;
padding: 0;
background: url(https://www.solluna.blog/wp-content/uploads/2023/02/bg.jpg) no-repeat center top #000000;
}
#effectimg {
position: fixed; /*描画固定*/
width: 100vw;
height: 100vh;
overflow: hidden;
}
function resizeCanvas() {
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
}
const canvas = document.getElementById("effectimg");
const ctx = canvas.getContext("2d");
const imgCnt = 40; // 画像の数 //
const aryImg = [];
const aryCloud = [];
const effectimgw = 2000; // canvasの横サイズ //
const effectimgh = 1000; // canvasの縦サイズ //
const imgBaseSizeW = 16; // 画像の横 //
const imgBaseSizeH = 20; // 画像の縦 //
const aspectMax = 2.5;
const aspectMin = 0.5;
const speedMax = 2.0; // 速度の最大値 //
const speedMin = 0.5; // 速度の最小値 //
const wind = 100;
const img = new Image();
img.src = "https://www.solluna.blog/wp-content/uploads/2023/02/sakura.png"; // 桜の画像を設定してください。//
img.onload = () => {
resizeCanvas();
flow_start();
};
function setImagas() {
let aspect = 0;
for (let i = 0; i < imgCnt; i++) {
aspect = Math.random() * (aspectMax - aspectMin) + aspectMin;
aryImg.push({
"posx": Math.random() * effectimgw,
"posy": Math.random() * effectimgh,
"sizew": imgBaseSizeW * aspect,
"sizeh": imgBaseSizeH * aspect,
"speedy": Math.random() * (speedMax - speedMin) + speedMin,
"angle": Math.random() * 360,
});
}
}
let idx = 0;
let idxc = 0;
let cos = 0;
let sin = 0;
const rad = Math.PI / 180;
function flow() {
ctx.clearRect(0, 0, effectimgw, effectimgh);
for (idx = 0; idx < imgCnt; idx++) {
aryImg[idx].posx += wind / aryImg[idx].sizew;
aryImg[idx].posy += aryImg[idx].speedy;
(idx % 2) ? aryImg[idx].angle += 1 : aryImg[idx].angle -= 1;
cos = Math.cos(aryImg[idx].angle * rad);
sin = Math.sin(aryImg[idx].angle * rad);
ctx.setTransform(cos, sin, sin, cos, aryImg[idx].posx, aryImg[idx].posy);
ctx.drawImage(img, 0, 0, aryImg[idx].sizew, aryImg[idx].sizeh);
ctx.setTransform(1, 0, 0, 1, 0, 0);
if (aryImg[idx].posy >= effectimgh) {
aryImg[idx].posy = -aryImg[idx].sizeh;
if (imgCnt < idx) {
aryImg.splice(idx, 1);
}
}
if (aryImg[idx].posx >= effectimgw) {
aryImg[idx].posx = -aryImg[idx].sizew;
if (imgCnt < idx) {
aryImg.splice(idx, 1);
}
}
}
for (idxc = 0; idxc < aryCloud.length; idxc++) {
ctx.drawImage(aryCloud[idxc].img, aryCloud[idxc].posx, aryCloud[idxc].posy, aryCloud[idxc].img.width, aryCloud[idxc].img.height);
aryCloud[idxc].posx += aryCloud[idxc].speed / 15;
if (aryCloud[idxc].posx > effectimgw) {
aryCloud[idxc].posx = -aryCloud[idxc].img.width;
}
}
}
function flow_start() {
setImagas();
setInterval(flow, 10);
}
実装方法
桜の画像を用意します。
画像に関しましてはご自身で制作していただくか、下記のリンクから画像を保存してご利用ください。
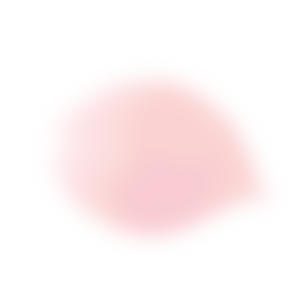
See the Pen 桜を降らせる by SOLLUNA (@ygi) on CodePen.
HTML
headタグの中にCSSを読み込みます。
<link rel="stylesheet" href="css/style.css">
bodyタグの中にcanvasを記載します。
<canvas id="sakura" width="2000px" height="1000px"></canvas>
body終了タグ直前にJSを読み込みます。
<script src="js/script.js"></script>
CSS
背景を設定します。
body {
margin: 0 auto;
padding: 0;
background: url(https://www.solluna.blog/wp-content/uploads/2023/02/bg.jpg) no-repeat top;
}
#effectimg {
position: fixed; /*描画固定*/
width: 100vw;
height: 100vh;
overflow: hidden;
}
JS
画像の数や落ちる速度や向きを調整します。
imgCnt
画像の数speedMax
速度の最大値speedMin
速度の最小値img.src
画像を設定
const canvas = document.getElementById("effectimg");
const ctx = canvas.getContext("2d");
const imgCnt = 40; // 画像の数 //
const aryImg = [];
const aryCloud = [];
const effectimgw = 2000; // canvasの横サイズ //
const effectimgh = 1000; // canvasの縦サイズ //
const imgBaseSizeW = 16; // 画像の横 //
const imgBaseSizeH = 20; // 画像の縦 //
const aspectMax = 2.5;
const aspectMin = 0.5;
const speedMax = 2.0; // 速度の最大値 //
const speedMin = 0.5; // 速度の最小値 //
const wind = 10;
const img = new Image();
img.src = "https://www.solluna.blog/wp-content/uploads/2023/02/sakura.png"; // 桜の画像を設定してください。//
応用編
葉っぱを降らせる
DEMOページをご覧ください。
See the Pen 葉っぱを降らせる by SOLLUNA (@ygi) on CodePen.
紅葉を降らせる
DEMOページをご覧ください。
See the Pen 紅葉を降らせる by SOLLUNA (@ygi) on CodePen.
雪を降らせる
DEMOページをご覧ください。